Python, a versatile and powerful programming language, owes much of its popularity to its simplicity and readability. Among its key features is the ability to define and use functions, which play a crucial role in structuring code and promoting reusability. Mastering Python functions is essential for any programmer looking to write efficient, maintainable, and scalable code. In this comprehensive guide, we will explore the fundamental concepts, advanced techniques, and best practices for working with Python functions.
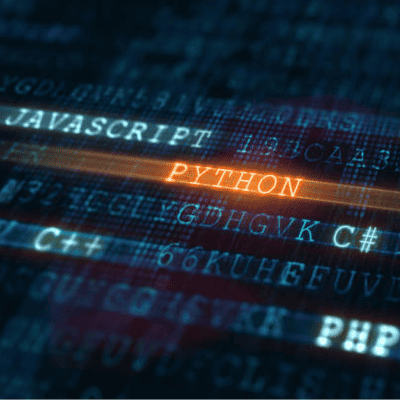
Understanding the Basics
1. Function Definition and Syntax:
At its core, a function in Python is a block of organized, reusable code designed to perform a specific task. Defining a function involves using the def
keyword followed by the function name and parameters. The function body is indented to indicate the code block belonging to the function.
2. Parameters and Arguments:
Parameters are variables that are used in a function’s definition, while arguments are the actual values passed to the function when it is called. Python supports different types of parameters, including positional, keyword, and default parameters.
3. Return Statements:
Functions can return values using the return
statement. If no return
statement is present, the function returns None
by default.
4. Scope and Lifetime of Variables:
Understanding variable scope is crucial when working with functions. Variables defined inside a function have local scope, and they are not accessible outside the function. Global variables, defined outside any function, have a broader scope.
Advanced Function Concepts
1. Lambda Functions:
Lambda functions, also known as anonymous functions, are concise one-liners defined using the lambda
keyword. They are handy for short operations and are often used in functional programming.
Mastering Python Functions: A Comprehensive Guide
Python, a versatile and powerful programming language, owes much of its popularity to its simplicity and readability. Among its key features is the ability to define and use functions, which play a crucial role in structuring code and promoting reusability. Mastering Python functions is essential for any programmer looking to write efficient, maintainable, and scalable code. In this comprehensive guide, we will explore the fundamental concepts, advanced techniques, and best practices for working with Python functions.
Understanding the Basics
1. Function Definition and Syntax:
At its core, a function in Python is a block of organized, reusable code designed to perform a specific task. Defining a function involves using the def
keyword followed by the function name and parameters. The function body is indented to indicate the code block belonging to the function.
pythonCopy code
def greet(name): print(f"Hello, {name}!")
2. Parameters and Arguments:
Parameters are variables that are used in a function’s definition, while arguments are the actual values passed to the function when it is called. Python supports different types of parameters, including positional, keyword, and default parameters.
pythonCopy code
def add_numbers(x, y=0): return x + y result = add_numbers(5, 3) # Positional arguments
3. Return Statements:
Functions can return values using the return
statement. If no return
statement is present, the function returns None
by default.
pythonCopy code
def square(x): return x ** 2 result = square(4) # Returns 16
4. Scope and Lifetime of Variables:
Understanding variable scope is crucial when working with functions. Variables defined inside a function have local scope, and they are not accessible outside the function. Global variables, defined outside any function, have a broader scope.
pythonCopy code
global_variable = 10 def my_function(): local_variable = 5 print(global_variable) # Accessible print(local_variable) # Accessible my_function() print(global_variable) # Accessible # print(local_variable) # Raises an error
Advanced Function Concepts
1. Lambda Functions:
Lambda functions, also known as anonymous functions, are concise one-liners defined using the lambda
keyword. They are handy for short operations and are often used in functional programming.
pythonCopy code
square = lambda x: x ** 2 result = square(5) # Returns 25
2. Decorators:
Decorators are a powerful and advanced feature in Python that allows the modification of the behavior of a function. They are defined using the @decorator
syntax and are commonly used for tasks such as logging, timing, and access control.
3. Closures:
Closures are functions that remember values in the enclosing scope even if they are not present in memory. They are created when a nested function references a variable from its containing function.
4. Generators:
Generators provide a convenient way to create iterators. They allow you to iterate over a potentially large sequence of data without loading the entire sequence into memory.
Best Practices for Function Design
1. Keep Functions Simple and Focused:
Functions should have a single responsibility and perform a specific task. Keeping functions small and focused enhances code readability and makes it easier to maintain.
2. Use Descriptive Names:
Choose meaningful names for your functions. A well-named function makes the code self-explanatory and reduces the need for excessive comments.
3. Avoid Global Variables:
Minimize the use of global variables, as they can lead to unintended side effects and make code harder to understand. Pass variables as parameters when possible.
4. Document Your Functions:
Provide clear and concise documentation for your functions. This includes describing the purpose of the function, expected input parameters, and the value it returns.
5. Handle Errors Gracefully:
Implement proper error handling within your functions. Use try-except blocks to catch and handle exceptions, ensuring that your code can gracefully recover from unexpected situations.
Testing and Debugging Functions
1. Unit Testing:
Writing unit tests for your functions is essential to ensure they behave as expected. Python provides the unittest
module for organizing and running test cases.
2. Debugging Tools:
Python comes with built-in debugging tools such as pdb
(Python Debugger). Inserting breakpoints in your code and using these tools can help identify and fix issues effectively.
Conclusion
Mastering Python functions is a key step toward becoming a proficient Python programmer. Whether you are a beginner or an experienced developer, understanding the basics, exploring advanced concepts, and following best practices will contribute to writing clean, maintainable, and efficient code. As you continue to refine your skills, consider applying these principles to real-world projects, collaborating with other developers, and staying updated with the Python ecosystem to leverage new features and improvements. Happy coding!
Recent Posts
If you're looking to start developing a mobile application and don't know what application OS to develop, then you've come to the right place. Among the leading platforms, Android and iOS dominate...
Knowing what IDE to use when you are just starting or planning to develop an app yourself is one of the common questions one might ask themselves. Advanced developers will just tell you that it's...