Python, a versatile and powerful programming language, is celebrated for its simplicity and readability. One of its key strengths lies in its control structures, which empower developers to direct the flow of a program. In this comprehensive guide, we will delve into the intricacies of Python’s control structures, exploring their types, applications, and best practices.
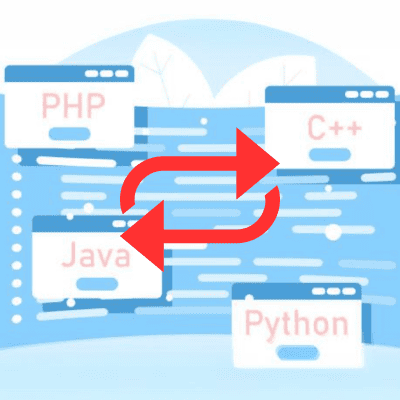
Understanding Control Structures
Control structures in Python are tools that allow developers to alter the execution flow of a program based on certain conditions. They include decision-making structures (if statements), looping structures (for and while loops), and branching structures (break and continue statements).
Conditional Statements: The Power of “if”
The if
statement is a fundamental control structure in Python, enabling developers to execute a block of code if a certain condition is met. For example:
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
As you can see from the code snippet, the “if” and “else” statements are used to determine if a variable has the necessary value to satisfy the conditions given in the statement which in this is case if x is greater than 5. This simple construct forms the basis for more complex decision-making processes within a program.
Looping Constructs: Iterating with Ease
Python provides two main looping constructs: for
and while
loops. Loops are very essential in making a code easier to write. It can be used in many cases and is very invaluable to a programmer’s tool belt.
The “for” Loop
The for
loop is ideal for iterating over a sequence (such as a list or a string) or a range of values. For instance:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
This loop iterates through the list of fruits, printing each one. The logic in this loop is that it will start printing out values in the array by starting to print out fruits[0] and each print it will add 1 to the index until there are no more values inside the array.
The “while” Loop
The while
loop continues executing a block of code as long as a specified condition remains true. Consider the following example:
count = 0
while count < 5:
print(count) count += 1
This loop prints numbers from 0 to 4, showcasing the continuous execution as long as the condition is satisfied. After each successful loop, the count will increase by 1 and thus nearing the condition given by the statement. We can also make infinite loops by making the condition be “true” and have to manually stop the while statement by using the break function which we will discuss next.
Branching Statements: Break and Continue
The “break” Statement
The break
statement is employed to exit a loop prematurely, regardless of the loop’s normal termination condition. This is useful when a certain condition is met, and further iterations are unnecessary.
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number == 3:
break print(number)
In this example, the loop terminates when the value 3 is encountered.
The “continue” Statement
On the other hand, the continue
statement skips the rest of the code within a loop for the current iteration and proceeds to the next iteration.
pythonCopy code
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number == 3:
continue print(number)
Here, the loop continues to the next iteration when the value 3 is encountered.
Switching to Switch: The Absence of Switch-Case
Unlike some programming languages, Python lacks a native switch
statement. However, this functionality can be emulated using dictionaries or if-elif-else constructs. While this approach may be less concise, it effectively achieves the same result.
def switch_case(case):
switch_dict = { "case1": "This is Case 1", "case2": "This is Case 2", "case3": "This is Case 3", }
return switch_dict.get(case, "This is the default case")
result = switch_case("case2") print(result)
In this example, the switch_case
function simulates a switch statement, returning the corresponding value for the given case.
Best Practices for Control Structures
- Code Readability: Python’s readability is one of its primary strengths. Write clear and concise code, using meaningful variable and function names to enhance comprehension.
- Avoid Nesting Too Deeply: Excessive nesting can make code hard to follow. Consider refactoring code with multiple nested structures to improve readability.
- Use List Comprehensions: Python’s list comprehensions offer a concise and readable way to create lists. Take advantage of them when appropriate.
- Consistent Indentation: Python relies on indentation to define blocks of code. Maintain consistent and readable indentation throughout your programs.
- Choose Appropriate Control Structures: Select the control structure that best fits the logic you want to implement. For example, use a
for
loop when iterating over a sequence and anif
statement for decision-making.
Conclusion
In this guide, we’ve explored the fundamental concepts of Python’s control structures, ranging from conditional statements and loops to branching constructs. Mastery of these elements is crucial for effective programming, enabling developers to create more dynamic and responsive applications.
As you continue your Python journey, remember that control structures are tools to shape the flow of your code. Use them wisely, prioritize readability, and unleash the full power of Python in your projects. Happy coding!
Recent Posts
If you're looking to start developing a mobile application and don't know what application OS to develop, then you've come to the right place. Among the leading platforms, Android and iOS dominate...
Knowing what IDE to use when you are just starting or planning to develop an app yourself is one of the common questions one might ask themselves. Advanced developers will just tell you that it's...